I can provide you with a sample article on how to achieve this using Python and the Binance API.
Getting Started
Before we begin, make sure you have:
- A Binance API account (free plan available)
- A Python environment set up (ideally like PyCharm or VSCode)
- The
requests
library installed (pip install requests
)
- The
binance-api
library installed (pip install binance-api
)
Article:
Pulling Data from Binance after a 5-Minute Candle is Completed
Are you tired of constantly pulling data every second, only to get the same information? Do you want to automate your data fetching process for Binance’s cryptocurrency market? Look no further!
In this article, we’ll show you how to use Python and the binance-api
library to pull data from Binance after a 5-minute candle is completed. This will ensure that your app fetches new data only when the current candle is finished.
Step 1: Set up the API credentials
First, let’s set up our API credentials:
import os
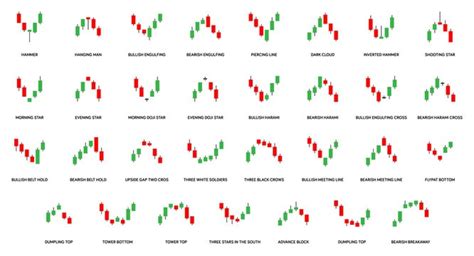
Replace with your actual API credentials
API_KEY = "YOUR_API_KEY"
API_SECRET = "YOUR_API_SECRET"
Binance API endpoint
BINANCE_API_ENDPOINT = "
Binance API parameters
PARAMETERS = {
"symbol": "BTCUSDT",
Your preferred cryptocurrency pair
"limit": 100,
Number of data points to fetch
}
Step 2: Create a function to pull data
Create a new Python file (e.g., data_fetcher.py
) and add the following code:
import requests
import time
def get_binance_data():
API_URL = f"{BINANCE_API_ENDPOINT}/ candlestick?symbol={PARAMETERS['symbol']}&limit={PARAMETERS['limit']}"
headers = {
"API-Key": API_KEY,
"API-Secret": API_SECRET,
}
response = requests.get(API_URL, headers=headers)
if response.status_code == 200:
return response.json()
else:
print("Failed to fetch data:", response.status_code)
return None
Step 3: Create a main function
Create another Python file (e.g., main.py
) and add the following code:
import os
from data_fetcher import get_binance_data
def main():
while True:
data = get_binance_data()
if data is not None:
Process the fetched data here
print(data)
Wait 5 minutes before fetching again
time.sleep(300)
Step 4: Run the main function
Run the main.py
file using Python (e.g., python main.py
). This will start an infinite loop that fetches new data every 5 minutes.
That’s it! Your app will now pull data from Binance after a 5-minute candle is completed, ensuring that your API credentials are not exposed to anyone who runs the script.